Some Simple JavaScript Functions
Monday, December 06, 2004 Some Simple JavaScript Functions by Mitchell Harper JavaScript can be one of the most useful additions to any web page. It comes bundled with Microsoft Internet Explorer and Netscape Navigator and it allows us to perform field validations, mouse-over images, open popup windows, and a slew of other things. If you have any experience with C, C++, PHP or Java, then you'll be glad to know that the syntax of JavaScript is very similar. In this article I will show you how to: Display the browser name and version number Change the text in the status bar of the browser Use an input box to get text from the user Use a message box to display text to the user Change the title of the browser window Before we can implement the functions i'm about to describe, we need to know how to setup our web page so that it can run the JavaScript. // JavaScript code goes here These script tags can be placed anywhere on the page, however it's common practice to place them between the and tags.
Page Events
Introduction If you place Javascript code into the head section of your page outside of functions then that Javascript will run before the web page content is loaded. If you place Javascript code outside of functions in the body section of your web page then that Javascript will run as the page is loading (when the loading of the page reaches the code). We can get Javascripts to run after the page has finished loading and even when the page is unloaded (by your visitor requesting another page) by attaching event handlers to the body tag itself. Onload The onload() event handler will run Javascript code that you want to run after everything on the page has finished loading. As you can see, in this example a parameter is being passed to the function that refers to some of the web page content. Onunload The other time in the life of a web page where you may want to run some Javascript is when the web page is being unloaded to make way for another web page. Onresize Onscroll A Better Way
Finding open tabs of reuse with delayedOpenTab (solved-fyi) • mo
I'm using the KB sample code to have my extension find a previously opened window via wm.getEnumerator("navigator:browser"). The sample code relies on (url == currentTab.currentURI.spec) to identify a tab for reuse. I need to perform a more complex matching and would like to achieve this my assigning addition content to the DOM document. Problem is that, when a new window is opened via recentWindow.delayedOpenTab(url) no result window is returned (addTab doesn't seem to execute at all). So- how can tabs be reused with more complex matching mechanisms than just URIs? Update: After some experiments (and especially looking at the QuickNote extension), I've found that I can open new tabs and assign data: Code: Select all var browserEnumerator = wm.getEnumerator('navigator:browser');var browserInstance = browserEnumerator.getNext().getBrowser(); var newTab = browserInstance.addTab(" "true");browserInstance.selectedTab = newTab; Ideas?
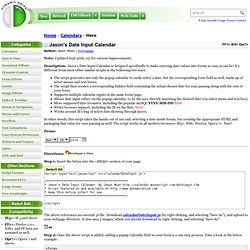
Jason's Date Input Calendar
Note: Updated Sept 20th, 05' for various improvements. Description: Jason's Date Input Calendar is designed specifically to make entering date values into forms as easy as can be! It's different from most other similar scripts in the following two ways: The script generates not only the popup calendar to easily select a date, but the corresponding form field as well, made up of select menus and text boxes. In other words, this script takes the hassle out of not only selecting a date inside forms, but creating the appropriate HTML and packaging that value for easy passing as well! Demo: Directions: Step 1: Insert the below into the <HEAD> section of your page: The above references an external .js file. Step 2: Once the above script is added, adding a popup Calendar field to your form is a one step process. <form> <script>DateInput('orderdate', true, 'DD-MON-YYYY')</script> The result is: Additional Information DateInput(DateName, Required*, DateFormat*, DefaultDate*) -Customizable variables
By Mark Kahn Many developers have a large library of JavaScript code at their fingertips that they developed, their collegues developed, or that they've pieced together from scripts all over the Internet. Have you ever thought that it would be nice to not have to search through all those files just to find that one function? This article will show you how dynamically include any JavaScript file, at runtime, by simply calling a function in that file! Here's an example: You have a function foo() in file bar.js. In your code, you know that foo() might be called, but it probably won't be because most people do not use its functionality. Dynamically Loading a Script As many developers know, there are at least two different ways to dynamically load a script at runtime. It is this second method that we're going to use, and we're going to exploit the fact that an XMLHTTP request has the capability to completely stall any script activity. First, some basics: how to create an XMLHTTP Object.
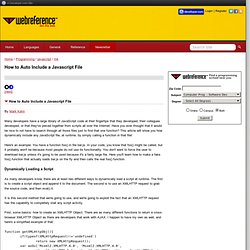
How to Auto Include a Javascript File - WebReference.com
Relying on DOM readiness to invoke a function (instead of window.onload)
Relying on DOM readiness to invoke a function (instead of window.onload) One of the most common event web developers check for before running their JavaScript is whether the page has completely loaded. For example: window.onload=function(){ walkmydog() } This technique has become very popular as a way of defining our scripts in a central location without having to worry about the availability of the page content it seeks to manipulate. Here's an demo of the technique we'll be looking at Checking DOM readiness in Firefox and Opera 9+ In Firefox and Mozilla, checking for DOM readiness and executing a function when it's ready is simple, as a special "DOMContentLoaded" keyword is supported that can be used in place of an element when calling document.addEventListener(): if (document.addEventListener) document.addEventListener("DOMContentLoaded", walkmydog, false) Checking DOM readiness in Internet Explorer In IE (includes IE7), there's no intrinsic way to detect DOM readiness.
JavaScript Kit- Your comprehensive JavaScript, DHTML, CSS, and Ajax stop
Online javascript beautifier
Raphaël—JavaScript Library
Dynamically populating a JavaScript image slideshow
Dynamically populating a JavaScript image slideshow A pesky problem with JavaScript slideshows it that you need to manually specify the images to display inside the script. If you have many images, this can quickly drive you up the wall. How about automating the process through PHP, by getting it to retrieve the file names of all images and passing it to your JavaScript slideshow to play around with? Lets get straight to it, by creating a PHP script that gets the file names of all images inside the directory the script is placed in, and return them as a JavaScript array: This script will grab the file names of every image in a directory (based on its extension) and output them as JavaScript array elements: where "dog.gif", "cat.gif" etc are the file names of the images inside the directory. Example: And there you have it!
There's nothing more troublesome than receiving orders, guestbook entries, or other form submitted data that are incomplete in some way. You can avoid these headaches once and for all with JavaScript's amazing way to combat bad form data with a technique called "form validation". The idea behind JavaScript form validation is to provide a method to check the user entered information before they can even submit it. JavaScript also lets you display helpful alerts to inform the user what information they have entered incorrectly and how they can fix it. In this lesson we will be reviewing some basic form validation, showing you how to check for the following: This lesson is a little long, but knowing how to implement these form validation techniques is definitely worth the effort on your part. This has to be the most common type of form validation. JavaScript Code: Working Example: Display: What we're doing here is using JavaScript existing framework to have it do all the hard work for us.
Form
Interactive Forms with Javascript, HTML Tutorial
Click here for Spanish Translation What are forms? One of the most important aspects of web design is getting information from the viewer to the webmaster. This is where HTML forms are used. If you have been on the internet, you have seen forms before. Google uses them for search queries, Amazon uses them for shipping and credit card information, your bank uses them for you to login. Why interactive forms? Forms are easy enough to create when they are simple, like search boxes. The problem I am going to use a business project as an example to teach interactive forms. Creating the interactive form We are going to create a page where you can enter the information for ordering flowers. <select id='numflowers'><option value='0'>Number of Flowers <option value='1'>1 <option value='2'>2 <option value='3'>3 <option value='4'>4 <option value='5'>5 <option value='6'>6 </select> This will create something looking like this: For this tutorial, I assume you have a basic knowledge of HTML. Javascript
Home
Sean Christmann has written another HTML5 Video and canvas demo that makes the video explode wherever it is clicked. Even when it is exploded, the pieces still continue to play the section of video that they were playing when the video was entirely together. After a few seconds, the pieces animate back together, reforming into a complete video once again. A very cool effect! One of the things that Sean had to do to get this implementation to run smoothly, was to have a second canvas act as a buffer to the final product. It is much faster to read from a canvas than it is from a video, so this caches every frame of video so that it doesn’t need to be retrieved as often. All very good information to know when writing your own crazy demos!
Badass JavaScript - Exploding HTML5 Video with JavaScript and Canvas
JavaScript Garden
Although JavaScript deals fine with the syntax of two matching curly braces for blocks, it does not support block scope; hence, all that is left in the language is function scope. function test() { // a scope for(var i = 0; i < 10; i++) { // not a scope // count } console.log(i); // 10} There are also no distinct namespaces in JavaScript, which means that everything gets defined in one globally shared namespace. Each time a variable is referenced, JavaScript will traverse upwards through all the scopes until it finds it. The Bane of Global Variables // script Afoo = '42'; // script Bvar foo = '42' The above two scripts do not have the same effect. Again, that is not at all the same effect: not using var can have major implications. // global scopevar foo = 42;function test() { // local scope foo = 21;}test();foo; // 21 Leaving out the var statement inside the function test will override the value of foo. // global scopevar items = [/* some list */];for(var i = 0; i < 10; i++) { subLoop();}
Demos below! As a sort-of reverse birthday present I’ve decided to release one of my largest projects, in recent memory. This is the project that I’ve been alluding to for quite some time now: I’ve ported the Processing visualization language to JavaScript, using the Canvas element. I’ve been working on this project, off-and-on now, for the past 7 months – it’s been a fun, and quite rewarding, challenge. The Processing Language The first portion of the project was writing a parser to dynamically convert code written in the Processing language, to JavaScript. It works “fairly well” (in that it’s able to handle anything that the processing.org web site throws at it) but I’m sure its total scope is limited (until a proper parser is involved). The language includes a number of interesting aspects, many of which are covered in the basic demos. Note: There’s one feature of Processing that’s pretty much impossible to support: variable name overloading. The Processing API Download How to Use Demos
Processing.js
JavaScript Garden
Eric Miraglia (@miraglia) is an engineering manager for the YUI project at Yahoo. Eric has been at Yahoo since 2003, working on projects ranging from Yahoo Sports to YUI. For the past several years, Eric and his colleagues on the YUI team have worked to establish YUI as the foundation for Yahoo’s frontend engineering work while open-sourcing the project and sharing it with the world under a liberal BSD license. Eric is an editor and frequent contributor to YUIBlog; his personal blog is at ericmiraglia.com. Prior to working at Yahoo, Eric taught writing at Stanford and elsewhere and led frontend engineering teams at several startups. Global variables are evil. Douglas Crockford has been teaching a useful singleton pattern for achieving this discipline, and I thought his pattern might be of interest to those of you building on top of YUI. 1. YAHOO.namespace("myProject"); This assigns an empty object myProject as a member of YAHOO (but doesn’t overwrite myProject if it already exists). 2.
A JavaScript Module Pattern
JavaScript ist eine Script-Sprache, die von Netscape entwickelt wurde. JavaScript-Programme sind in den HTML-Code integriert und können diesen steuern. Hierzu ist ein JavaScript-fähiger Browser erforderlich, der die Anweisungen JavaScript-Programm unmittelbar ausführt (interpretiert). Man kann damit z.B. die Eingabewerte von Formularen prüfen oder verarbeiten, witere Fenster öffnen u.v.a. JavaScript ist objektbasiert, d.h. der Programmierer kann auf die Objekte des Browsers, deren Eigenschaften und Methoden zugreifen und sogar neue Objekte erzeugen (aber keine neuen Klassen definieren). Sie finden hier einige kommentierte Beispiele und die Scripts: Zum Download Weitere JavaScripts: Astronomie mit JavaScript Sonnenstand, Tabellen Sonnenauf- und -untergang, Zeitzonen u.v.a.m. Getestet mit: What is JavaScript? JavaScript is a scripting language created by Netscape Communications. One thing to remember is that JavaScript is not an alternative to Java, but a complement to it. Home
JavaScript-Beispiele
Moment.js - A lightweight javascript date library
nanoScroller.js
nanoScroller.js is a jQuery plugin that offers a simplistic way of implementing Mac OS X Lion-styled scrollbars for your website. It uses minimal HTML markup being .nano > .nano-content. The other scrollbar div elements .pane > .nano-slider are added during run time to prevent clutter in templating. The latest version utilizes native scrolling and works with the iPad, iPhone, and some Android Tablets. Downloads To start using, you need three basic things: 1. The following type of markup structure is needed to make the plugin work: <div id="about" class="nano"><div class="nano-content"> ... content here ... Copy the HTML markup. Link to the nanoscroller.css file inside your page's <head> section (...or copy the contents from it to your page's main stylesheet file). You should specify a width and a height to your container, and apply some custom styling for your scrollbar. 3. Running this script will apply the nanoScroller plugin to all DOM elements with a .nano className. Advanced methods
Online javascript beautifier
Run javascript onload but not in body tag ??
Documentation - JavaScript API (beta)
Expanding Text Areas Made Elegant
Essential JavaScript Design Patterns For Beginners
Learning Javascript with Object Graphs
Script Junkie | How to Write Maintainable OO JavaScript Code
Introduction to Object-Oriented JavaScript
A re-introduction to JavaScript (JS Tutorial)
Top news - Echo JS
unconed/MathBox.js
Book of Modern Front-end Tooling
JavaScript Allongé
John Resig - JavaScript Programmer
JS: The Right Way - Iceweasel
JSbooks - free javascript books - Iceweasel
[javascript #mvc] Closure Tools - Google Code
[javascript #mvc] CodeBrief
JavaScript Tutorial
JavaScript Tutorial
JavaScript Language Reference