I have recently been drinking the jQuery kool aid. One of my favorite plugins for it is the excellent Datepicker plugin , which I am using whenever I want to display a nice calendar to users. Last week I needed it to work on a single selectbox that listed available dates but couldn't find any documentation for it. There are some examples of a datepicker for multiple selectboxes (day/month/year), but not when you only have a single one. I am using the jQuery UI 1.5 beta release , so your milage may vary since the API has changed quite a bit lately. The Markup The example I am working with has the following structure: Select date May 1, 2008 May 3, 2008 May 5, 2008 May 25, 2008 Select date May 1, 2008 May 3, 2008 May 5, 2008 May 25, 2008 Datepicker primarily works with input elements so you will need to add a hidden element for it. I prefer to have the value as an ISO date string and then use more user friendly format that is visible so I tend to do this anywhere the UI is important. return {};
wtf.hax: jQuery DatePicker and Selectbox
Event Pooling with jQuery Using Bind and Trigger: Managing Compl
Managing Complexity in the UI As everyone knows, the more dependencies you have in a system, the harder maintaining that system is. Javascript is no exception- and orchestrating actions across complex user interfaces can be a nightmare if not done properly. Luckily, there’s a great pattern for orchestrating complex interaction in a disconnected way. Problems with the Observer Pattern The observer pattern is great for some things, but it still requires a dependency between the observer and the subject. observer pattern Event Pooling Event pooling is simply a variation on the Observer pattern, where a “middle man” is used to orchestrate the publish/subscribe system. Event Pool This provides some cool functionality- especially because if you need to reference the subject (called the publisher), you can get a direct reference via the event pool. Show me Code! We are going to do two things: First, update a span tag to show an address when a user enters a name, city, or state. and from html:
jQuery for Designers - Tutorials and screencasts
Building an interactive map with jQuery instead of Flash - New M
At the end of last year, we launched a website for Coastal & Marine Sciences in North Carolina with one of our Agency Partners, Liaison Design Group. As a part of the project, the website was supposed to have an interactive map that showed some information and the location of the various Marine Science outposts across the state. The locations would be represented by dots and upon clicking one an info box would pop up and display information about the location. To make the map as engaging as possible, there needed to be smooth animations and crisp graphics. Traditionally such a project would require the use of Flash. We try to avoid Flash whenever possible, so I began to consider how the project could be accomplished in jQuery. There were a number of advantages of using jQuery over Flash for the project: Updates were Easy: Since the map was going to be a visual representation of html, it would be easy to update the data with our Content Management Software. The Five Locations Conclusion
jQuery: The Write Less, Do More, JavaScript Library
jQuery plugin: Tablesorter 2.0
Author: Christian Bach Version: 2.0.5 (changelog) Licence: Dual licensed (just pick!)under MIT or GPL licenses. Please with sugar on top! Don't hotlink the tablesorter.js files. Update! Helping out! Comments and love letters can be sent to: christian@tablesorter.comchristian at tablesorter dot com. tablesorter is a jQuery plugin for turning a standard HTML table with THEAD and TBODY tags into a sortable table without page refreshes. tablesorter can successfully parse and sort many types of data including linked data in a cell. Multi-column sorting Parsers for sorting text, URIs, integers, currency, floats, IP addresses, dates (ISO, long and short formats), time. TIP! To use the tablesorter plugin, include the jQuery library and the tablesorter plugin inside the <head> tag of your HTML document: tablesorter works on standard HTML tables. Start by telling tablesorter to sort your table when the document is loaded: $(document).ready(function() { $("#myTable").tablesorter(); } ); NOTE!
Ketchup Plugin
Ketchup is a small (3.4KB minified & gzipped) jQuery Plugin that helps you to validate your forms. Out of the box it has 18 basic validations and a bubble like style. But truly this Plugin wants to be hacked to fit your needs. Easily write your own validations and overwrite/extend the default behaviour. Bubbles are not for everyone... Default Behavior If you like the style of the bubbles and all validations you need are already included you can get this Plugin up and running like so: Your HTML Header Include the default stylesheet (located in . <! Your HTML By default Ketchup checks the data-validate attribute of form fields if it can find matching validations. Your Javascript Just call ketchup() on your form, voilà. $('#default-behavior').ketchup(); Declare fields to validate in the call In last version Ketchup checked the class attribute for validations... which was not everyones taste because class should be used for defining CSS classes. Validate on different events Included Validations
jQuery for Designers - Tutorials and screencasts
Martin Angelov HTTP is a stateless protocol, which means that every request you make to a website is standalone and therefore cannot keep data by itself. But this simplicity is also one of the reasons for its widespread adoption in the early ears of the web. There is, however, a way to keep information between requests in the form of cookies. This way you can have effective session managemet and persistent data. There are two ways to work with cookies – server side (PHP, ASP etc) and client side (JavaScript). Cookies and PHP Setting cookies To create a cookie in PHP, you need to use the setcookie function. setcookie( 'pageVisits', $visited, time()+7*24*60*60, '/', 'demo.tutorialzine.com' ); If you pass 0 as an expiration time (which is the default behavior) the cookie will be lost on browse restart. There are two additional parameters that you could pass to the function, which are not given here. For most practical purposes, you would only need the first four parameters, omitting the rest.
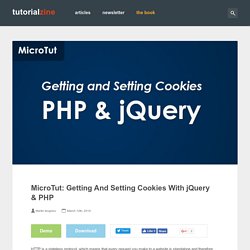
MicroTut: Getting And Setting Cookies With jQuery & PHP – Tutori
Here's 10 tips that will makes you code more efficiently with jQuery. 1. Be lazy // Don't if ( $ ( '#item' ). get ( 0 )) { $ ( '#item' ). someFunction (); } // Or if ( $ ( '#item' ). length ) { $ ( '#item' ). someFunction (); } // Just do $ ( '#item' ). someFunction (); jQuery will call the function if there is a match, no need to double check. 2. // You can but.. $ ( document ). ready ( function (){ // ... }); // There is a shorter equivalent $ ( function (){ // ... }); It should be well known, but obviously it is not. 3. // Don't $ ( '#frame' ). fadeIn (); $ ( '#frame .title' ). show (); $ ( '#frame a:visited' ). hide ; // Do $ ( '#frame' ). fadeIn () . find ( '.title' ). show (). end () . find ( 'a:visited' ). hide (); Unnecessary DOM traversal is a expensive operation, avoid it when possible. 4. 5. 6. Using jQuery's each and map is more reliable because they won't break if another library is extending the Array object. 7. Events can be namespaced The method also accept namespaces Instead of
blog / 10 useful jQuery authoring tips
Description A jQuery plugin from GSGD to give advanced easing options. Please note, the easing function names changed in version 1.2. Please also note, you shouldn't really be hotlinking the script from this site, if you're after a CDN version you could do worse than try cdnjs.com Download Download the following: Example Click on any of the yellow headers to see the default easing method in action (I've set as easeOutBounce for the demo, just because it's obviously different). Select easing types for the demo first one for down, second one for up. The Clicker Updates 12/11/07 1.3 jQuery easing now supports a default easing mode. 04/10/07 1.2 Updated to include all methods from Robert Penners easing equations. 28/06/07 1.1.1 Updated the method to not overwrite the newly renamed 'swing', or the new 'linear' style coming in 1.1.3. 22/06/07 Rewritten the above to include callback syntax, nothing else has changed. Advertisements Need reliable hosting for your blog? Credits Donate Usage Default Custom
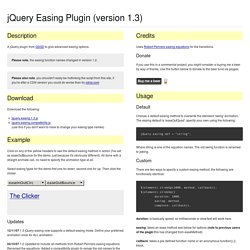
jQuery Easing Plugin
Hover above and feel for yourself, the nifty effect of Lava Lamp. What you just experienced is nothing but the LavaLamp menu packaged as a plugin for the amazing jQuery javascript library. I personally believe that the effect rivals that of flash – Don’t you? Especially considering the fact that it is extremely light weight. Just so you know, it weighs just 700 bytes(minified)! Often I have noticed, that the credits are usually granted towards the end. As User Interface developers, we know that one of the first widgets our visitors use is a “Menu”. I hope you agree that a typical HTML widget consists of 3 distinct components. A semantically correct HTML markupA CSS to skin the markupAn unobstrusive javascript that gives it a purpose Now lets follow the above steps and implement the LavaLamp menu for your site. Step 1: The HTML Since most UI developers believe that an unordered list(ul) represents the correct semantic structure for a Menu/Navbar, we will start by writing just that. Bonus
LavaLamp for jQuery lovers! | Ganesh
jQuery Mobile
Here we developed several frequently-used jquery ui plugins. They are all free (open source LGPL license), easy to use, and with great functionalities. Hope you can enjoy them. You may see them all in action JQuery Event Calendar Plugin jquery event calendar wdCalendar is a jquery based google calendar clone. Day/week/month view provided.create/update/remove events by drag & drop.Easy way to integrate with database.All day event/more days event provided. See it in action | Check documentation | download JQuery Scroll Tab Plugin jquery scroll tab wdScrollTab is a tab panel which has ability to scroll for tabs that do not fit on the page. croll tab in case of too many tabs.dynamical/remove hide.rich javascript API See it in action | Check documentation | download JQuery Checkbox Tree Plugin jquery checkbox tree wdTree is lightweight jQuery plugin for present tree with nested check boxes. parents/children checkinglazy load from database configurable node attributes JQuery Date Picker Plugin
JQuery Plugins | jquery plugins
La carte de l'Europe cliquable en Css et jQuery
Un truc particulièrement utile ! Au lieu de faire ça : $(document).ready(function() { } Vous pouvez faire ceci, qui revient au même : L'attribut target n'est pas valide en (X)HTML strict. Du coup, nous utiliserons l'attribut rel et un peu de jQuery pour créer un attribut dynamiquement et éviter les erreurs de validation. $('a[rel=external]').attr('target','_blank'); <a href=" rel="external">Queness dans une nouvelle fenêtre</a> Cette astuce est très pratique lorsque vous faites des menus avec des balises <ul>. $("ul li").click(function(){ window.location=$(this).find("a").attr("href"); return false;}); Vous voulez donner la possibilité de modifier le design de votre site ? $("a.cssSwitcher").click(function() { $('link[rel=stylesheet]').attr('href' , $(this).attr('rel'));}) Certains veulent désactiver le clic droit ou créer leur propre menu contextuel. $(document).bind("contextmenu",function(e){ return false; }); Ce script affichera les coordonnées x et y de la souris.
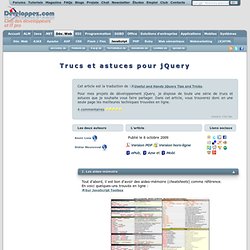
Trucs et astuces pour jQuery - Club des décideurs et professionn
List of Really Useful Plugins For jQuery Developers
jQuery – The “write less, do more” JavaScript library is great for rapid web development. New components, tools and applications are literally popping up on daily basis. Numerous plugins are also available to help jQuery developers. Following list of jQuery plugins is different from other lists which usually features UI components . PeriodicalUpdater Adds ability to loads content at specified intervals, and if the content being pulled doesn’t change, it gradually increases interval to saves bandwidth and reduce the CPU usage on the client’s machine.BlockUI Lets you simulate synchronous behavior when using AJAX, it will prevent user activity with the page (or part of the page) until it is deactivated. Animation & Effects Charts & Graphs jqPlot Pure JavaScript plotting plugin for creating client side charts and graphsGoogle Charts Wrapper for Google API charts. Design Files Fonts Forms iPhone Flick Adds Apple iPod/iPhone (firmware 2.0+) flick/scroll functionality to jQuery. Maps Mouse
The Publish Subscribe or pub/sub pattern is used to logically decouple object(s) that generate an event, and object(s) that act on it. It is a useful pattern for object oriented development in general and especially useful when developing asynchronous Javascript applications. This post explores its implementation in jQuery. The Dojo javascript framework provides an explicit topic-based mechanism for “publishing” and “subscribing” to events. Peter Higgins, Dojo’s author, has also written a jQuery plugin that replicates this functionality. jQuery custom events provide a built in means to use the publish subscribe pattern in a way that is functionally equivalent, and syntactically very similar, to Higgin’s pub/sub plugin: Just bind observers to document. $(document).bind("eventType", function() { alert(" I <3 Fanny"}); var obj = new MyObject(); $(obj).trigger("eventType"); // nothing obj.ownerDocument = document; $(obj).trigger("eventType"); // I <3 Fanny!
Publish/Subscribe with jQuery Custom Events – Publish/Subscribe with jQuery Custom Events – Bocoup Web Log
GMAP3 – Plugin jQuery – L’API Google Map v.3 Integration à la sauce jQuery
Aujourd’hui je vais vous parler d’un autre plugin jQuery : GMAP3. Un plugin qui va vous permettre d’utiliser l’API Google Map v3 Integration via le framework javascript jQuery. Ce qui facilite l’utilisation de l’API de Google pour les habitués de jQuery. J’ai découvert ce plugin grâce à jb, un lecteur du blog (cf. ici) et je l’en remercie. Voici la mise en place de base du plugin : Tout d’abord l’appel des différentes librairies à utiliser : Puis le code, qui va permettre d’insérer dans la div #test, la map qui pointera “la place de l’étoile à Paris”, avec un tooltip positionné dessus, avec un texte d’exemple : “Texte Exemple”. A savoir que tous les éléments de l’API de Google Map sont utilisable et disponible via GMAP3. Bon code à tous Articles qui peuvent vous intéresser :
SmartUpdater est un plugin jQuery permettant de lancer une requête Ajax périodiquement sur son serveur. Très pratique si vous souhaitez mettre en place des interfaces dynamiques nécessitant un refresh de données régulières à partir de votre serveur. L'idée du plugin est de simplifier le processus d'appel périodique du serveur et de permettre de le contrôler facilement. Voici un exemple d'utilisation du plugin: 1.$("#example1").smartupdater({ 2. url : 'gettime_json.php', 3. dataType:'json', 4. minTimeout: 5000 5. }, function (data) { 6. Dans cet exemple, nous allons initialiser un appel à notre serveur sur le fichier PHP gettime_json.php toutes les 5 secondes, spécifier que l'on souhaite un retour en JSON (Pour simplifier le traitement du résultat), puis dès que nous recevons une réponse du serveur, nous mettons à jour l'heure reçue par le serveur. Grâce au plugin, vous pourrez utiliser plusieurs méthodes pour contrôler à tout moment votre timer: Site Officiel
Smartupdater - Interrogez périodiquement votre serveur avec jQuery
Tweetable 1.6 launched | web dev & design blog
jQuery Slideshow Carousel 1 of 2
jQuery Easy Slides v1.1 - Possibly the easiest to use jQuery plugin for making slideshows
10 Useful jQuery Plugins for Images
24 Fresh and Useful jQuery Plugins Worth Checking Out | DesignBeep
Tiny Circleslider: A lightweight jQuery plugin
20 (More) Fresh jQuery Image Gallery/Slider Plugins and Tutorials Worth a Look | DesignBeep
jQuery Tooltip Plugin Demo
jQuery Flash Plugin - Basic Example
headjs.com
jQuery Mobile Masterclass: Build a Simple, Attractive Twitter App for iPhone
Best jQuery Plugins of 2011
SP, jQuery, and FullCalendar—Now with SPS
jQuery Accordion Tutorial
20+ Superb jQuery Plugins | jQuery
Articles: Web Design and Development Tutorials and Ideas | Elated.com
Zoomooz.js
Isotope
jQuery Geo
Online Ticket Booking System using ASP.NET
jQuery | ilovecolors - Part 2
How to Make a Smooth Animated Menu with jQuery