Ruby Basic Tutorial
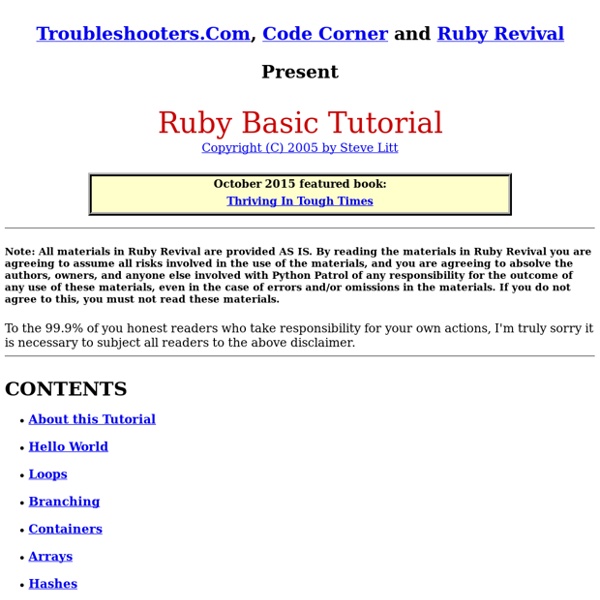
Troubleshooters.Com, Code Corner and Ruby Revival Present Ruby Basic Tutorial Copyright (C) 2005 by Steve Litt Note: All materials in Ruby Revival are provided AS IS. By reading the materials in Ruby Revival you are agreeing to assume all risks involved in the use of the materials, and you are agreeing to absolve the authors, owners, and anyone else involved with Python Patrol of any responsibility for the outcome of any use of these materials, even in the case of errors and/or omissions in the materials. If you do not agree to this, you must not read these materials. To the 99.9% of you honest readers who take responsibility for your own actions, I'm truly sorry it is necessary to subject all readers to the above disclaimer. CONTENTS This is a Ruby tutorial for one not knowing Ruby. Ruby can be used as a fully object oriented language, in which case you'd create classes and objects to accomplish everything. This is the simplest possible Ruby program, hello.rb. Let's count to 10... Retry
Ruby in Twenty Minutes
What if we want to say “Hello” a lot without getting our fingers all tired? We need to define a method! irb(main):010:0> def hiirb(main):011:1> puts "Hello World!"irb(main):012:1> end=> :hi The code def hi starts the definition of the method. The Brief, Repetitive Lives of a Method Now let’s try running that method a few times: irb(main):013:0> hiHello World! Well, that was easy. What if we want to say hello to one person, and not the whole world? irb(main):015:0> def hi(name)irb(main):016:1> puts "Hello #{name}!" So it works… but let’s take a second to see what’s going on here. Holding Spots in a String What’s the #{name} bit? irb(main):019:0> def hi(name = "World")irb(main):020:1> puts "Hello #{name.capitalize}!" A couple of other tricks to spot here. Evolving Into a Greeter What if we want a real greeter around, one that remembers your name and welcomes you and treats you always with respect. The new keyword here is class. So how do we get this Greeter class set in motion?
Ruby, Ruby on Rails, and _why: The disappearance of one of the world’s most beloved computer programmers
Illustration by Charlie Powell. In March 2009, Golan Levin, the director of Carnegie Mellon University’s interdisciplinary STUDIO for Creative Inquiry, invited an enigmatic and famed computer programmer known to the virtual world only as “Why the Lucky Stiff” or “_why”—no, not a typo—to speak at a CMU conference called Art && Code—also not a typo—an event where artsy nerds and nerdy artists gather to talk shop. _why came to Pittsburgh and presented his latest project to a room full of a student programmers and artists. He was scruffily handsome, seemingly in his early- to mid-30s, with shaggy brown hair falling in his eyes and a constant half-smile. At this symposium, he wore a pair of oversize sunglasses and a tidy sports coat with a red pocket square, a silly riff on a stuffy professor’s outfit. He riffed on his nom d’Internet, Why the Lucky Stiff: “Some people want to call me Mr. Back in the old days, you could hack your Commodore 64 without too much trouble. Why? That’s it.
Ruby on Rails Tutorial: Learn Rails by Example book and screencasts by Michael Hartl
Michael Hartl Contents Foreword My former company (CD Baby) was one of the first to loudly switch to Ruby on Rails, and then even more loudly switch back to PHP (Google me to read about the drama). Though I’ve worked my way through many Rails books, this is the one that finally made me “get” it. The linear narrative is such a great format. Enjoy! Derek Sivers (sivers.org) Founder, CD Baby Acknowledgments The Ruby on Rails Tutorial owes a lot to my previous Rails book, RailsSpace, and hence to my coauthor Aurelius Prochazka. I’d like to acknowledge a long list of Rubyists who have taught and inspired me over the years: David Heinemeier Hansson, Yehuda Katz, Carl Lerche, Jeremy Kemper, Xavier Noria, Ryan Bates, Geoffrey Grosenbach, Peter Cooper, Matt Aimonetti, Gregg Pollack, Wayne E. About the author Michael Hartl is the author of the Ruby on Rails Tutorial, the leading introduction to web development with Ruby on Rails. Copyright and license Welcome to the Ruby on Rails Tutorial.
Related:
Related: