Read JavaScript Allongé
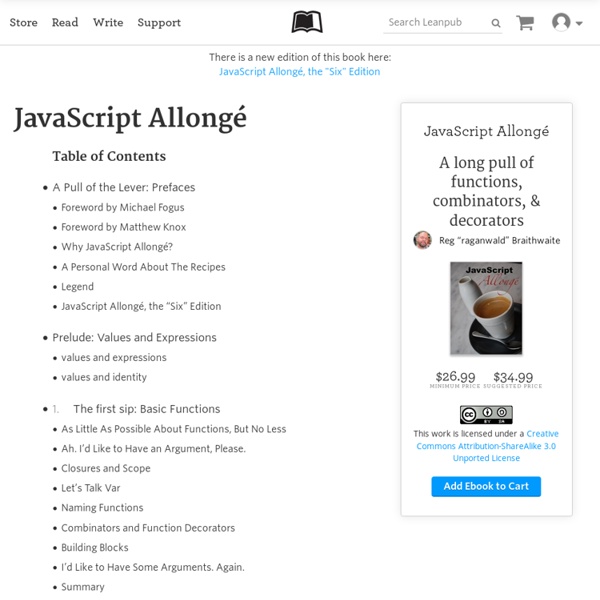
A Pull of the Lever: Prefaces “Café Allongé, also called Espresso Lungo, is a drink midway between an Espresso and Americano in strength. There are two different ways to make it. Foreword by Michael Fogus As a life-long bibliophile and long-time follower of Reg’s online work, I was excited when he started writing books. The act of writing is an iterative process with (very often) tight revision loops. In the case of JavaScript Allongé, you’ll find the Leanpub model a shining example of effectiveness. As a staunch advocate of functional programming, much of what Reg has written rings true to me. Enjoy. – Fogus, fogus.me Foreword by Matthew Knox A different kind of language requires a different kind of book. JavaScript holds surprising depths–its scoping rules are neither strictly lexical nor strictly dynamic, and it supports procedural, object-oriented (in several flavors!) –Matthew Knox, mattknox.com Why JavaScript Allongé? how the book is organized A Personal Word About The Recipes Instead of:
Writing Jasmine unit tests in ES6
Previously I showed how to write application code using ES6, but wouldn't it be nice to use ES6 when writing unit tests as well? In the following post I will demonstrate how to write Jasmine tests using ES6 syntax. Setting up the test environment is fairly easy, but to move things along I've included my sample package.json configuration package.json I will be using Karma to run the tests, so the next step is to add a karma.config.js file to your project. karma.config.js I have chosen to run my tests using PhantomJS – a headless browser ideal for these types of tasks. Babel is used for transpiling ES6 to ES5. The last bit of plumbing is to add test-context.js to your project: test-context.js var context = require.context('. This is where you tell the test runner where your files are located. context.keys contains an array of test files. Now that the environment is bootstrapped it's time to write some tests! To keep it simple we will be writing tests for a simple calculator implementation. Sources
ECMAScript 6: New Features: Overview and Comparison
"A good programming language is a conceptual universe for thinking about programming." — Alan J. Perlis Constants Support for constants (also known as "immutable variables"), i.e., variables which cannot be re-assigned new content. ECMAScript 6 — syntactic sugar: reduced | traditional const PI = 3.141593; PI > 3.0; ECMAScript 5 — syntactic sugar: reduced | traditional Scoping Block-Scoped Variables Block-scoped variables (and constants) without hoisting. for (let i = 0; i < a.length; i++) { let x = a[i]; …}for (let i = 0; i < b.length; i++) { let y = b[i]; …} let callbacks = [];for (let i = 0; i <= 2; i++) { callbacks[i] = function () { return i * 2; };} callbacks[0]() === 0; callbacks[1]() === 2; callbacks[2]() === 4; Block-Scoped Functions Block-scoped function definitions. { function foo () { return 1; } foo() === 1; { function foo () { return 2; } foo() === 2; } foo() === 1;} Arrow Functions Expression Bodies More expressive closure syntax. Statement Bodies Lexical this Extended Parameter Handling
Raynos/chain-stream
Task.js: Beautiful Concurrency for JavaScript
Related:
Related: